TelegraphChat
Chat informations are stored in database inside a telegraph_chats
table and can be retrieved using DefStudio\Telegraph\Models\TelegaphChat
model or using a custom Chat model.
Custom Chat Model
To customize on your own Chat model, make sure that your custom model extends the DefStudio\Telegraph\Models\TelegraphChat
, e.g. App\Models\Chat
, it will looks like this:
<?php namespace App\Models; use DefStudio\Telegraph\Models\TelegraphChat as BaseModel; class Chat extends BaseModel { }
You should specify the class name of your model in the models.chat
key of the telegraph config file.
'models' => [ 'chat' => App\Models\Chat::class, ],
Available methods
info()
Retrieves the chat data from telegram
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->info(); /* id: xxxxx type: group title: my telegram group ... */
memberCount()
Retrieves the chat member count from telegram
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->memberCount(); /* 42 */
memberInfo()
Retrieves the chat member info from telegram
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->memberInfo('user_id'); /* status: string user: { id: xxxxx firstName: string lastName: string ... } */
Optional parameters
Attachments methods only supports required parameters, optional parameters can be sent through Telegraph ->withData()
method:
$telegraphChat->message('hi')->withData('caption', 'test')->send(); $telegraphChat->withData('caption', 'test')->message('hi')->send();
Custom Thread
Attachments can be sent to a specific Thread through Telegraph ->inThread()
method:
$telegraphChat->message('hi')->inThread(thread_id)->send();
message()
Starts a Telegraph
call to send a message
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->message('hello!')->send();
html()
Starts a Telegraph
call to send a message using html formatting
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->html('<b>hello</b>')->send();
markdown()
Starts a Telegraph
call to send a message using markdown formatting
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->markdown('*hello*')->send();
markdownV2()
Starts a Telegraph
call to send a message using markdown V2 formatting
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->markdownV2('*hello*')->send();
edit()
Starts a Telegraph
call to edit a message
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->edit($messageId)->message('new text')->send();
editCaption()
Starts a Telegraph
call to edit an attachment's caption
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->editCaption($messageId)->message('new caption')->send();
editMedia()
Starts a Telegraph
call to edit a media messages with a new media content
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->editMedia($messageId)->photo($path)->send(); $telegraphChat->editMedia($messageId)->document($path)->send(); $telegraphChat->editMedia($messageId)->animation($path)->send(); $telegraphChat->editMedia($messageId)->video($path)->send(); $telegraphChat->editMedia($messageId)->audio($path)->send();
replaceKeyboard()
Starts a Telegraph
call to replace a message keyboard (see keyboards for details)
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->replaceKeyboard( $messageId, Keyboard::make()->buttons([ Button::make('open')->url('https://test.dev') ]) )->send();
deleteKeyboard()
Starts a Telegraph
call to remove a message keyboard (see keyboards for details)
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->deleteKeyboard($messageId)->send();
WarningFollow installation instructions for creating the database tables
deleteMessage()
Starts a Telegraph
call to delete a message
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->deleteMessage($messageId)->send();
deleteMessages()
Starts a Telegraph
call to delete multiple messages
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->deleteMessages(array $messageIds)->send();
forwardMessage()
forwards a message from another chat
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->forwardMessage($fromChat, $messageId)->send();
pinMessage()
Starts a Telegraph
call to pin a message
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->pinMessage($messageId)->send();
unpinMessage()
Starts a Telegraph
call to unpin a message
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->pinMessage($messageId)->send();
unpinAllMessages()
Starts a Telegraph
call to unpin all messages
/** @var \DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->unpinAllMessages()->send();
action()
Tells the chat users that something is happening on the bot's side. The status is set for up to 5 seconds or when a new message is received from the bot.
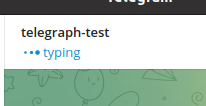
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->action(ChatActions::TYPING)->send();
setBaseUrl()
allows to override Telegram API url on a per-message basis:
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->setBaseUrl('https://my-secret-server.dev')->message('secret message')->send();
setTitle()
sets chat title
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->setTitle("my chat")->send();
setDescription()
sets chat description
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->setDescription("a test chat with my bot")->send();
setMessageReaction()
changes the chosen reactions on a message
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->setMessageReaction($messageId, ['type' => 'emoji', 'emoji' => '👍'])->send();
reactWithEmoji()
reaction on a message with emoji
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->reactWithEmoji($messageId, '👍')->send();
reactWithCustomEmoji()
reaction on a message with custom emoji
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->reactWithCustomEmoji($messageId, '12312')->send();
setChatPhoto()
sets chat profile photo
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->setChatPhoto(Storage::path('photo.jpg'))->send();
generatePrimaryInviteLink()
generates a new primary invite link for a chat. Any previously generated primary link is revoked. For more info, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->generatePrimaryInviteLink()->send();
createInviteLink()
creates an additional invite link for a chat. For more info about options, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->createInviteLink() ->name('September promotional link') //optional ->expire(today()->addMonth()) //optional ->memberLimit(42) //optional ->withJoinRequest() //optional ->send();
editInviteLink()
edits an existing invite link for a chat. For more info about options, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->editInviteLink('http://t.me/123456') ->name('new name') //optional ->expire(today()->addYear()) //optional ->memberLimit(12) //optional ->withJoinRequest(false) //optional ->send();
revokeInviteLink()
revokes an existing invite link for a chat. For more info, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->revokeInviteLink('http://t.me/123456')->send();
setPermissions()
set users permissions for a chat. For more info, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->setPermissions([ ChatPermissions::CAN_INVITE_USERS, ChatPermissions::CAN_CHANGE_INFO, ChatPermissions::CAN_ADD_WEB_PAGE_PREVIEWS => true, ChatPermissions::CAN_SEND_MESSAGES => false, ])->send();
approveJoinRequest()
approve an user join request to the chat
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->approveJoinRequest($userid)->send();
declineJoinRequest()
decline an user join request to the chat
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->declineJoinRequest($userid)->send();
banMember()
ban a user in a group, a supergroup or a channel. In the case of supergroups and channels, the user will not be able to return to the chat on their own using invite links. For more info, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->banMember($userId) ->until(now()->addDay()); //optional, only for supergroups and channels ->andRevokeMessages() //optional, always true for supergroups and channels ->send();
unbanMember()
unban a user in a group, a supergroup or a channel. For more info, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->unbanMember($userId, true)->send();
restrictMember()
restrict a user in a group, a supergroup or a channel from taking the give actions. For more info, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->restrictMember($userId[ DefStudio\Telegraph\Enums\ChatPermissions::CAN_PIN_MESSAGES => false, DefStudio\Telegraph\Enums\ChatPermissions::CAN_INVITE_USERS => true, DefStudio\Telegraph\Enums\ChatPermissions::CAN_SEND_MESSAGES, ]) ->until(now()->addDay()) //optional+ ->send();
promoteMember()
promotes a user in a group, a supergroup or a channel to administrator status. For more info, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->promoteMember($userid, [ DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_PIN_MESSAGES => false, DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_INVITE_USERS => true, DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_CHANGE_INFO, ]) ->send();
demoteMember()
demote a user in a group, a supergroup or a channel from administrator status.
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->demoteMember($userid)->send();
poll
creates a native poll. For more info, see telegram bot documentation
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->poll("What's your favourite programming language?") ->option('php') ->option('typescript') ->option('rust') ->allowMultipleAnswers() ->validUntil(now()->addMinutes(5)) ->send();
menuButton
Retrieves chat menu button
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $button = $telegraphChat->menuButton()->send();
setMenuButton
set chat menu button
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->setMenuButton()->default()->send(); //restore default $telegraphChat->setMenuButton()->commands()->send(); //show bot commands in menu button $telegraphChat->setMenuButton()->webApp("Web App", "https://my-web.app")->send(); //show start web app button
Attachments
invoice()
sends an invoice
$telegraphChat->invoice('Invoice title') ->description('Invoice Description') ->currency('EUR') ->addItem('Item Label', 10) ->send();
document()
sends a document
$telegraphChat->document($documentPath)->send();
location()
sends a location attachment
$telegraphChat->location(12.345, -54.321)->send();
photo()
sends a photo
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->photo(Storage::path('photo.jpg'))->send();
voice()
sends a vocal message
use DefStudio\Telegraph\Models\TelegraphChat; /** @var TelegraphChat $telegraphChat */ $telegraphChat->voice(Storage::path('voice.ogg'))->send();
quiz
creates a quiz. For more info, see telegram bot documentation
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->quiz("What's your favourite programming language?") ->option('php', correct: true) ->option('typescript') ->option('rust') ->explanation('We all love php, right?') ->validUntil(now()->addMinutes(5)) ->send();
dice
An animated emoji attachment that will display a random value can be sent through Telegraph ->dice()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->dice()->send();
animation
An animation attachment can be sent through Telegraph ->animation()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->animation()->send();
video
A video attachment can be sent through Telegraph ->video()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->video()->send();
audio
An audio attachment can be sent through Telegraph ->audio()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->audio()->send();
sticker
A sticker attachment can be sent through Telegraph ->sticker()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->sticker()->send();
venue
A Venue attachment can be sent through Telegraph ->venue()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->venue(10, 10, 'title', 'address')->send();
media group
Group of photos, videos, documents or audios as an album can be sent through Telegraph ->mediaGroup()
method:
$telegraphChat->mediaGroup([ [ 'type' => 'photo', 'media' => 'https://my-repository/photo1.jpg', ], [ 'type' => 'photo', 'media' => 'https://my-repository/photo2.jpg', ] ])->send();
Forum Topic
create topic
A Forum Topic can be created through Telegraph ->createForumTopic()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->createForumTopic('topic name', 7322096, 'emoji_id')->send();
edit topic
A Forum Topic can be edited through Telegraph ->editForumTopic()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->editForumTopic(123456, 'new name', 'emoji_id')->send();
close topic
A Forum Topic can be closed through Telegraph ->closeForumTopic()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->closeForumTopic(123456)->send();
reopen topic
A Forum Topic can be reopened through Telegraph ->reopenForumTopic()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->reopenForumTopic(123456)->send();
delete topic
A Forum Topic can be deleted through Telegraph ->deleteForumTopic()
method:
/** @var DefStudio\Telegraph\Models\TelegraphChat $telegraphChat */ $telegraphChat->deleteForumTopic(123456)->send();