Chat API calls
chatAction()
Tells the chat users that something is happening on the bot's side. The status is set for up to 5 seconds or when a new message is received from the bot.
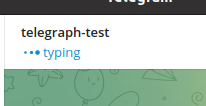
Telegraph::chatAction(ChatActions::TYPING)->send();
deleteMessage()
deletes a message
Telegraph::deleteMessage($messageId)->send();
deleteMessages()
deletes multiple messages
Telegraph::deleteMessages(array $messageIds)->send();
forwardMessage()
forwards a message from another chat
Telegraph::forwardMessage($fromChat, $messageId)->send();
pinMessage()
pins a message
Telegraph::pinMessage($messageId)->send();
unpinMessage()
unpins a message
Telegraph::unpinMessage($messageId)->send();
unpinAllMessages()
unpin al messages
Telegraph::unpinAllMessages()->send();
deleteKeyboard()
removes a message keyboard (see keyboards for details)
Telegraph::deleteKeyboard($messageId)->send();
replaceKeyboard()
replace a message keyboard (see keyboards for details)
Telegraph::replaceKeyboard( $messageId, Keyboard::make()->buttons([ Button::make('open')->url('https://test.dev') ]) )->send();
setTitle()
sets chat title
Telegraph::setTitle("my chat")->send();
setDescription()
sets chat description
Telegraph::setDescription("a test chat with my bot")->send();
setMessageReaction()
changes the chosen reactions on a message
Telegraph::setMessageReaction($messageId, ['type' => 'emoji', 'emoji' => '👍'])->send();
reactWithEmoji()
reaction on a message with emoji
Telegraph::reactWithEmoji($messageId, '👍')->send();
reactWithCustomEmoji()
reaction on a message with custom emoji
Telegraph::reactWithCustomEmoji($messageId, '12312')->send();
setChatPhoto()
sets chat profile photo
chatInfo()
retrieves Chat data from Telegram APIs
Telegraph::chatInfo()->send(); /* id: xxxxx type: group title: my telegram group ... */
setChatMenuButton()
Set menu button. For detailed info, see docs here and here
Telegraph::setChatMenuButton()->default()->send(); //restore default Telegraph::setChatMenuButton()->commands()->send(); //show bot commands in menu button Telegraph::setChatMenuButton()->webApp("Web App", "https://my-web.app")->send(); //show start web app button
Warningif no chat is active when calling this, the default bot's menu button will be changed.
chatMenuButton()
retrieves a bot current menu button info
Telegraph::chatMenuButton()->send();
chatMemberCount()
retrieves Chat member count
Telegraph::chatMemberCount()->send();
chatMember()
retrieves a Chat member
Telegraph::chatMember($userId)->send();
chatAdministrators()
retrieves a list of administrators in a chat, which aren't bots
Telegraph::chatAdministrators()->send();
userProfilePhotos()
retrieves the User's profile photos
Telegraph::userProfilePhotos($userId)->send();
generateChatPrimaryInviteLink()
generates a new primary invite link for a chat. Any previously generated primary link is revoked. For more info, see telegram bot documentation
Telegraph::generateChatPrimaryInviteLink()->send();
createChatInviteLink()
creates an additional invite link for a chat. For more info about options, see telegram bot documentation
Telegraph::createChatInviteLink() ->name('September promotional link') //optional ->expire(today()->addMonth()) //optional ->memberLimit(42) //optional ->withJoinRequest() //optional ->send();
editChatInviteLink()
edits an existing invite link for a chat. For more info about options, see telegram bot documentation
Telegraph::editChatInviteLink('http://t.me/123456') ->name('new name') //optional ->expire(today()->addYear()) //optional ->memberLimit(12) //optional ->withJoinRequest(false) //optional ->send();
revokeChatInviteLink()
revokes an existing invite link for a chat. For more info, see telegram bot documentation
Telegraph::revokeChatInviteLink('http://t.me/123456')->send();
setChatPermissions()
set users permissions for a chat. For more info, see telegram bot documentation
Telegraph::setChatPermissions([ ChatPermissions::CAN_INVITE_USERS, ChatPermissions::CAN_CHANGE_INFO, ChatPermissions::CAN_ADD_WEB_PAGE_PREVIEWS => true, ChatPermissions::CAN_SEND_MESSAGES => false, ])->send();
approveChatJoinRequest()
approve an user join request to the chat
Telegraph::approveChatJoinRequest($userid)->send();
declineChatJoinRequest()
decline an user join request to the chat
Telegraph::declineChatJoinRequest($userid)->send();
banChatMember()
ban a user in a group, a supergroup or a channel. In the case of supergroups and channels, the user will not be able to return to the chat on their own using invite links. For more info, see telegram bot documentation
Telegraph::banChatMember($userid) ->until(now()->addDay()); //optional, only for supergroups and channels ->andRevokeMessages() //optional, always true for supergroups and channels ->send();
unbanChatMember()
unban a user in a group, a supergroup or a channel. For more info, see telegram bot documentation
Telegraph::unbanChatMember($userid)->send();
restrictChatMember()
restrict a user in a group, a supergroup or a channel from taking the give actions. For more info, see telegram bot documentation
Telegraph::restrictChatMember($userid, [ DefStudio\Telegraph\Enums\ChatPermissions::CAN_PIN_MESSAGES => false, DefStudio\Telegraph\Enums\ChatPermissions::CAN_INVITE_USERS => true, DefStudio\Telegraph\Enums\ChatPermissions::CAN_SEND_MESSAGES, ]) ->until(now()->addDay()) //optional ->send();
promoteChatMember()
promotes a user in a group, a supergroup or a channel to administrator status. For more info, see telegram bot documentation
Telegraph::promoteChatMember($userid, [ DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_PIN_MESSAGES => false, DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_INVITE_USERS => true, DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_CHANGE_INFO, ]) ->send();
demoteChatMember()
demote a user in a group, a supergroup or a channel from administrator status.
Telegraph::demoteChatMember($userid)->send();