Telegram API calls
answerInlineQuery
send back the results for an inline query
Telegraph::answerInlineQuery($inlineQuery->id(), [ InlineQueryResultPhoto::make($logo->id."-light", "https://logofinder.dev/$logo->id/light.jpg", "https://logofinder.dev/$logo->id/light/thumb.jpg") ->caption('Light Logo'), InlineQueryResultPhoto::make($logo->id."-dark", "https://logofinder.dev/$logo->id/dark.jpg", "https://logofinder.dev/$logo->id/dark/thumb.jpg") ->caption('Dark Logo'), ])->cache(seconds: 600)->send();
botInfo
retrieves Bot data from Telegram APIs
Telegraph::botInfo()->send(); /* id: xxxxx is_bot: true first_name: telegraph-test username: my_test_bot can_join_groups: true can_read_all_group_messages: false supports_inline_queries: false */
botUpdates
retrieves the bot updates from Telegram APIs
Telegraph::bot($telegraphBot)->botUpdates()->send();
WarningManual updates polling is not available if a webhook is set up for the bot. Webhook should be remove first using its unregisterWebhook method
Long Polling
In production environment, a timeout (in seconds) should be declared, in order to allow long polling:
Telegraph::bot($telegraphBot)->botUpdates(timeout: 60)->send();
chatAction
Tells the chat users that something is happening on the bot's side. The status is set for up to 5 seconds or when a new message is received from the bot.
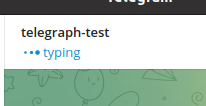
Telegraph::chatAction(ChatActions::TYPING)->send();
deleteMessage
deletes a message
Telegraph::deleteMessage($messageId)->send();
deleteMessages
deletes multiple messages
Telegraph::deleteMessages(array $messageIds)->send();
forwardMessage
forwards a message from another chat
Telegraph::forwardMessage($fromChat, $messageId)->send();
pinMessage
pins a message
Telegraph::pinMessage($messageId)->send();
unpinMessage
unpins a message
Telegraph::unpinMessage($messageId)->send();
unpinAllMessages
unpin al messages
Telegraph::unpinAllMessages()->send();
deleteKeyboard
removes a message keyboard (see keyboards for details)
Telegraph::deleteKeyboard($messageId)->send();
document
sends a document
Telegraph::document($documentPath)->send();
edit
edits a message
Telegraph::edit($messageId)->markdown('new message')->send();
editCaption
edits an attachment caption
Telegraph::editCaption($messageId)->markdownV2('new caption')->send();
editMedia
edits a media messages with a new media content
Telegraph::editMedia($messageId)->photo($path)->send(); Telegraph::editMedia($messageId)->document($path)->send(); Telegraph::editMedia($messageId)->animation($path)->send(); Telegraph::editMedia($messageId)->video($path)->send(); Telegraph::editMedia($messageId)->audio($path)->send();
getWebhookDebugInfo
retrieves webhook debug data for the active bot
$response = Telegraph::getWebhookDebugInfo()->send();
location
sends a location attachment
Telegraph::location(12.345, -54.321)->send();
Contact
sens a contact attachment
Telegraph::contact('3331122111', 'firstName')->send();
markdown
compose a new telegram message (parsed as markdown)
Telegraph::markdown('*hello* world')->send();
markdownV2
compose a new telegram message (parsed as markdownV2)
Telegraph::markdownV2('*hello* world')->send();
message
compose a new telegram message (will use the default parse mode set up in config/telegraph.php
)
Telegraph::message('hello')->send();
html
compose a new telegram message (parsed as html)
Telegraph::html('<b>hello</b> world')->send();
photo
sends a photo
Telegraph::photo($pathToPhotoFile)->send();
registerBotCommands
register commands in Telegram Bot in order to display them to the user when the "/" key is pressed
Telegraph::registerBotCommands([ 'command1' => 'command 1 description', 'command2' => 'command 2 description' ])->send();
getRegisteredCommands
retrieve bot's registered commands.
$response = Telegraph::getRegisteredCommands()->send(); $response->json('result');
registerWebhook
register a webhook for the active bot
Telegraph::registerWebhook()->send();
replaceKeyboard
replace a message keyboard (see keyboards for details)
Telegraph::replaceKeyboard( $messageId, Keyboard::make()->buttons([ Button::make('open')->url('https://test.dev') ]) )->send();
replyWebhook
replies to a webhook callback
Telegraph::replyWebhook($callbackQueryId, 'message received', $showAlert)->send();
store
Downloads a media file and stores it in the given path
/** @var DefStudio\Telegraph\DTO\Photo $photo */ Telegraph::store($photo, Storage::path('bot/images'), 'The Photo.jpg');
unregisterBotCommands
resets Telegram Bot registered commands
Telegraph::unregisterBotCommands()->send();
unregisterWebhook
unregister a webhook for the active bot
Telegraph::registerWebhook()->send();
voice
sends a vocal message
Telegraph::voice($pathToVoiceFile)->send();
when
allows to execute a closure when the given condition is verified
Telegraph::when(true, fn(Telegraph $telegraph) => $telegraph->message('conditional message')->send());
setBaseUrl
allows to override Telegram API url on a per-message basis:
Telegraph::setBaseUrl('https://my-secret-server.dev')->message('secret message')->send();
setTitle
sets chat title
Telegraph::setTitle("my chat")->send();
setDescription
sets chat description
Telegraph::setDescription("a test chat with my bot")->send();
setChatPhoto
sets chat profile photo
chatInfo
retrieves Chat data from Telegram APIs
Telegraph::chatInfo()->send(); /* id: xxxxx type: group title: my telegram group ... */
setChatMenuButton
Set menu button. For detailed info, see docs here and here
Telegraph::setChatMenuButton()->default()->send(); //restore default Telegraph::setChatMenuButton()->commands()->send(); //show bot commands in menu button Telegraph::setChatMenuButton()->webApp("Web App", "https://my-web.app")->send(); //show start web app button
Warningif no chat is active when calling this, the default bot's menu button will be changed.
chatMenuButton
retrieves a bot current menu button info
Telegraph::chatMenuButton()->send();
getFileInfo
Retrieve file info from ID
Telegraph::getFileInfo($fileId)->send();
chatMemberCount
retrieves Chat member count
Telegraph::chatMemberCount()->send();
chatMember
retrieves a Chat member
Telegraph::chatMember($userId)->send();
userProfilePhotos
retrieves the User's profile photos
Telegraph::userProfilePhotos($userId)->send();
generateChatPrimaryInviteLink
generates a new primary invite link for a chat. Any previously generated primary link is revoked. For more info, see telegram bot documentation
Telegraph::generateChatPrimaryInviteLink()->send();
createChatInviteLink
creates an additional invite link for a chat. For more info about options, see telegram bot documentation
Telegraph::createChatInviteLink() ->name('September promotional link') //optional ->expire(today()->addMonth()) //optional ->memberLimit(42) //optional ->withJoinRequest() //optional ->send();
editChatInviteLink
edits an existing invite link for a chat. For more info about options, see telegram bot documentation
Telegraph::editChatInviteLink('http://t.me/123456') ->name('new name') //optional ->expire(today()->addYear()) //optional ->memberLimit(12) //optional ->withJoinRequest(false) //optional ->send();
revokeChatInviteLink
revokes an existing invite link for a chat. For more info, see telegram bot documentation
Telegraph::revokeChatInviteLink('http://t.me/123456')->send();
setChatPermissions
set users permissions for a chat. For more info, see telegram bot documentation
Telegraph::setChatPermissions([ ChatPermissions::CAN_INVITE_USERS, ChatPermissions::CAN_CHANGE_INFO, ChatPermissions::CAN_ADD_WEB_PAGE_PREVIEWS => true, ChatPermissions::CAN_SEND_MESSAGES => false, ])->send();
banChatMember
ban a user in a group, a supergroup or a channel. In the case of supergroups and channels, the user will not be able to return to the chat on their own using invite links. For more info, see telegram bot documentation
Telegraph::banChatMember($userid) ->until(now()->addDay()); //optional, only for supergroups and channels ->andRevokeMessages() //optional, always true for supergroups and channels ->send();
unbanChatMember
unban a user in a group, a supergroup or a channel. For more info, see telegram bot documentation
Telegraph::unbanChatMember($userid)->send();
restrictChatMember
restrict a user in a group, a supergroup or a channel from taking the give actions. For more info, see telegram bot documentation
Telegraph::restrictChatMember($userid, [ DefStudio\Telegraph\Enums\ChatPermissions::CAN_PIN_MESSAGES => false, DefStudio\Telegraph\Enums\ChatPermissions::CAN_INVITE_USERS => true, DefStudio\Telegraph\Enums\ChatPermissions::CAN_SEND_MESSAGES, ]) ->until(now()->addDay()) //optional ->send();
promoteChatMember
promotes a user in a group, a supergroup or a channel to administrator status. For more info, see telegram bot documentation
Telegraph::promoteChatMember($userid, [ DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_PIN_MESSAGES => false, DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_INVITE_USERS => true, DefStudio\Telegraph\Enums\ChatAdminPermissions::CAN_CHANGE_INFO, ]) ->send();
demoteChatMember
demote a user in a group, a supergroup or a channel from administrator status.
Telegraph::demoteChatMember($userid)->send();
poll
creates a native poll. For more info, see telegram bot documentation
Telegraph::poll("What's your favourite programming language?") ->option('php') ->option('typescript') ->option('rust') ->allowMultipeAnswers() ->validUntil(now()->addMinutes(5)) ->send();
quiz
creates a quiz. For more info, see telegram bot documentation
Telegraph::quiz("What's your favourite programming language?") ->option('php', correct: true) ->option('typescript') ->option('rust') ->explanation('We all love php, right?') ->validUntil(now()->addMinutes(5)) ->send();
dump
print a dump()
of the current api call status for testing purposes
Telegraph::message('test')->dump();
dd
print a dd()
of the current api call status for testing purposes
Telegraph::message('test')->dd();
withData
set custom Telegraph data attribute
Telegraph::withData('key', 'value');